March 23, 2025
SDK 43
New Engine features:
- Added support for game controllers (Xbox, DualSense, DualShock, Nintendo Pro Controller, and generic joysticks) across all platforms.
- Improved physics engine plugin integration.
- Introduced a debug visualization API for enhanced real-time debugging.
- Implemented an Internal Color dialog for a more streamlined UI experience.
- Major improvements to the Explorer.
- Explorer has two icon sets for best icons visualization over different monitor DPIs.
- Executor and Explorer update the Controller class for automatic controller support.
- Explorer now supports multiple control info callbacks (allowing body info to be displayed within node info).
- Basic physics visualization (shapes and joints) has been added to Explorer.
- Inertia tensor information is now available in Explorer.
- All runtime parameter editors have been refactored to support right-click editing, expanding the range of editable types.
- Explorer has been migrated to the Internal Color dialog.
- Scale parameter has been added to the MeshAnimation import Explorer extension.
- The MeshPrimitive extension now correctly handles internal shapes during object editing.
- ScriptFlow and MaterialFlow extensions now use the Internal Color dialog.
- Improved geometry combination performance in the Mesh tool.
- Added 12 Hello Controller manual sample.
- Added tests/system/desktop for the Desktop class.
- Added tests/system/controller for the Controller class.
- Added new physics samples for each physics plugin (Box2D, Bullet, Jolt, PhysX), including 6dof, friction, hinge, impulse, restitution, shapes, and slider.
- Added scripts/script_node_flow_controller, a sample demonstrating flow-based virtual controller animation based on real controller events.
- Added scripts/script_node_flow_keyboard, a sample showcasing flow-based node control using window keyboard events.
- Introduced a new interface/color plugin for controls-based color dialog support.
- Box2D now supports capsule and cylinder shapes.
- The Box2D plugin has been updated to the latest Box2D library.
- The Jolt plugin has been updated to the latest Jolt library.
- All physics plugins now support engine-driven parameter updates, including friction, forces, impulses, shapes, and joints.
- Physics plugins now provide real-time velocity values to the engine.
- The default number of ControlFlow types has been increased to 512.
- ControlCallback has been added to ControlFlow to support custom color dialogs.
- PolyHaven and Sketchfab plugins now reduce preview image brightness when a modal control is present.
- ScriptFlow now includes guards to prevent assertion failures caused by invalid parameters (e.g., getting a child by index).
- The ControlFlow node dialog now displays the current node parameter value.
- The number of Tellusim::Function<> template arguments has been increased to 9.
- ControlRoot now includes a mouse offset parameter, allowing callback handlers to evaluate control offset/scale values when embedded within a ControlArea.
- Added a ‘disabled modal state’ option to ControlRoot, allowing modal focus without dimming other controls.
- Introduced new Mesh|BrepNode|Node|ObjectNode|Material::findChild|getChild() methods that accept literal name arguments.
- Introduced a movable flag for ControlDialog to restrict movement.
- Added InsideCallback to ControlRect for non-rectangular control shapes.
- Added MatrixNxM::outer() product operator.
- Implemented math operations between MatrixNxM types, including addition, subtraction, and scalar multiplication.
- RenderDraw now supports debug primitives visualization, including lines, quadratics, cubics, arrows, rectangles, quads, circles, boxes, spheres, cylinders, and capsules.
- Added a bias parameter to RenderDraw, allowing controllable depth overlap during rendering.
- BodyRigid axes locks are now controlled by BodyRigid flags instead of dedicated methods.
- Added BodyRigid::FlagKeepAwake that prevents Body from sleeping.
- Added BodyRigid::addForce(), addTorque(), addLinearImpulse(), and addAngularImpulse().
- BodyRigid::updateMass() now recalculates body mass and inertia during the next update.
- Added control over maximum linear and angular velocities for BodyRigid.
- Each SceneGraph entity now includes is*Updated() flags for better interoperations.
- Game Controller support has been added for Windows, Linux, macOS, Android, and iOS. On Windows, the system uses GameInput if available, falling back to XInput by default.
- The quat_to_mat4x3() function has been added to SceneMath.shader
- The get_body_transform() function has been added to SceneBodies.shader
- The get_num_graph_nodes() and get_graph_base_node() functions have been added to SceneGraphs.shader
- Introduced the base_scene_body member to the RenderFrame structure for accessing all body parameters.
- Tangent-space generation performance dramatically increased for meshes with same normals (giant planes).
- PrefixScan and RadixSort now correctly detect the presence of FlagIndirect for dispatchIndirect() operations.
- Corrected joint body assignment during all scene clone operations (nodes, bodies, graphs, scenes).
- Joystick events have been added to the Android activity.
- Corrected window and DialogMenu icon creation on Windows.
- Improved retina-aware DialogMenu icon rendering on macOS.
- New macOS devices have been added to Info class.
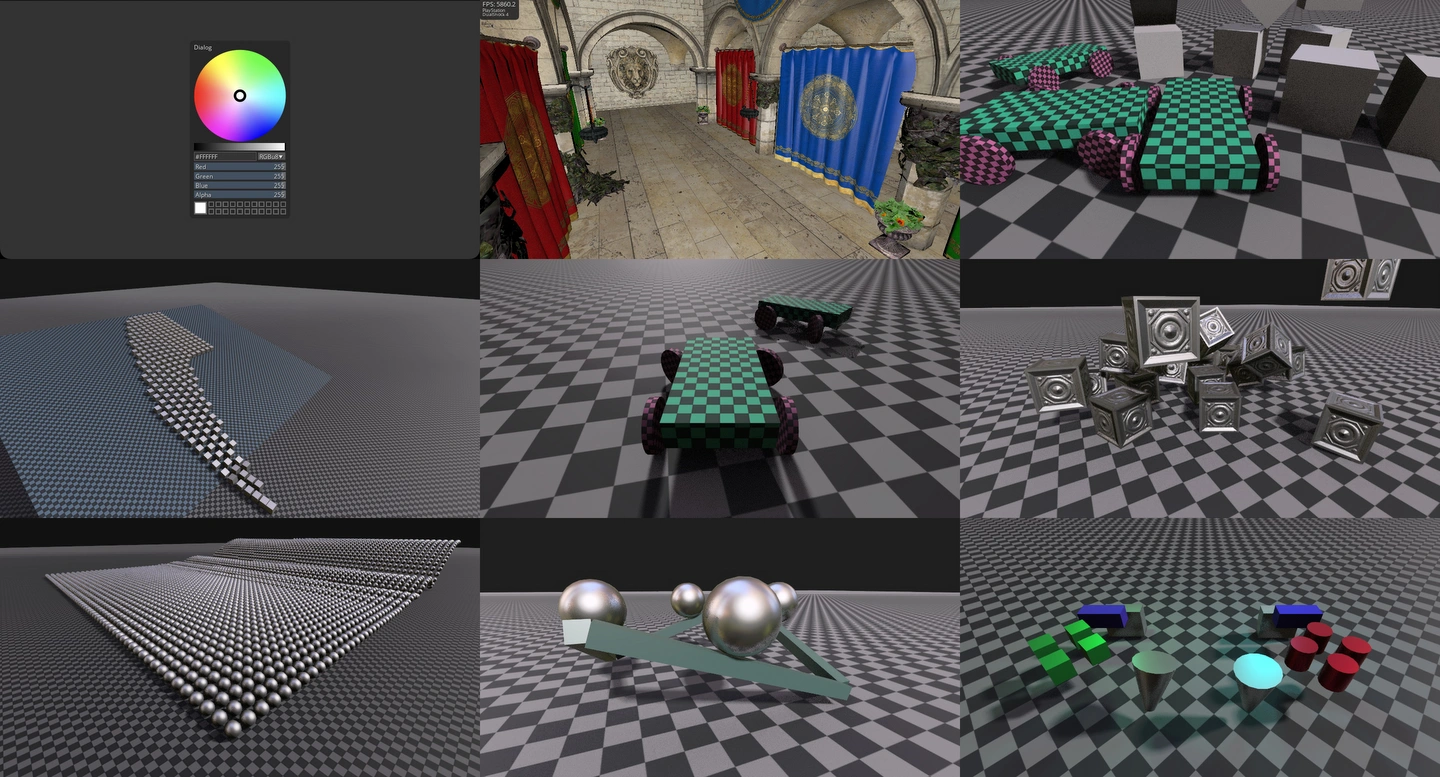
February 23, 2025
SDK 42
New Engine features:
- Introduced node-based API scripting.
- Added support for an unlimited number of mesh morph targets.
- Correct deformed morph geometries tracing.
- Fixed correct normal calculations for non-uniformly scaled objects when using the non-uniform material option.
- MeshAnimation Explorer extension for animation importing and retargeting has been added.
- SceneScriptFlow Explorer extension, which enables ScriptFlow functionality, has been added.
- ScenePrefab extension with Scattering and all Physics plugins has been added to the Explorer.
- Fragment and Compute app templates have been added to the project tool.
- MaterialFlow iridescent and marble material samples.
- Object mesh morph sample.
- Many new ScriptFlow samples have been added.
- ScriptFlow plugin now offers node-based Tellusim API scripting.
- More socket shapes have been added to ControlFlow.
- ControlFlow type masks now support up to 384 types.
- ControlElement has a new color multiplier parameter.
- Many new nodes have been added to the MaterialFlow plugin.
- Physics plugins support dynamic gravity changes.
- Physics plugins automatically handle body/shape/joint removals.
- Reflection plugin provides information about enums and callbacks.
- ARLink plugin (client to the Tellusim ARLink app) enables real-time facial animation.
- New MeshAttribute::TypeIndex attribute type acts as an index to the optimized (source) position attribute. Morph target attributes can be stored in a compact form and accessed via this index.
- Control of the ControlSplit size is now managed within the ControlRoot interface.
- Pressed callback has been added for ControlSlider.
- ObjectMesh::FlagSkeleton transforms the parent NodeObject into the source skeleton for all child nodes (e.g., different clothes on a character).
- ObjectAnimation::setEmbedded()|isEmbedded() flag controls animation embedding within the scene.
- ControlTree now returns the currently focused item for tooltips.
- Expressions now accept non-default matrix names.
- Trigonometric operations have been added for Vector<> types.
- Script scene classes can now store source code inside scene files.
- Object::createLocal()|GlobalTransforms() methods have been added for simple hierarchical node operations.
- A dedicated morph target buffer has been added to ObjectMesh.
- geometry/TellusimLine.h provides line-related math utilities.
- math/TellusimNoise.h provides Perlin and fractal noise samplers.
- Internal FBX loader now supports blend shapes.
- Updated mesh file format effectively compresses blend targets by storing only modified data.
- Default camera’s ZFar clip plane is Maxf32.
- Default point light radius is 100.
- Matrix4x3f type has been added to the Expression parser.
- Corrected skinned NodeObject bounding box visualization.
- Script::setParameter(), Material::setUniform(), and Material::setTexture() now no longer cause assertions when an invalid name is provided.
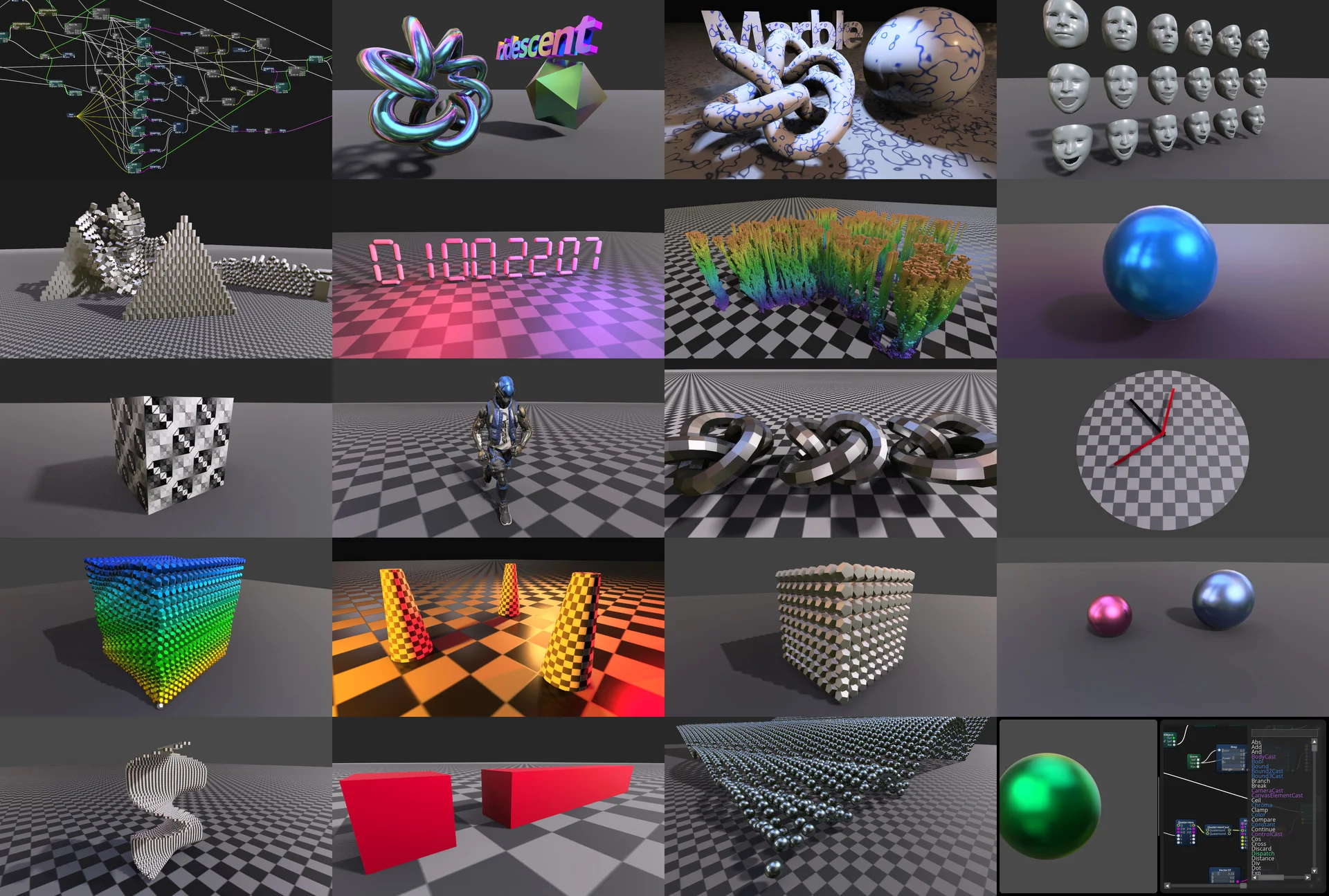
January 20, 2025
SDK 41
New Engine features:
- C++ reflection.
- JavaScript API.
- Node-based materials editing.
- Material depth intersection blending.
- Randomized texture coordinate sampling.
- All Core SDK language examples render torii objects.
- C++ API reflection plugin has been added.
- JavaScript API plugin for JavaScript-only applications has been added.
- MaterialFlow plugin provides full node-based material editing functionality.
- webp Image format plugin has been added.
- Bound types have been added to all languages.
- Flow plugin includes base GLSL generation library.
- Flow plugin supports different variants and versions.
- Input and output attachment, and create callbacks have been added to the Flow plugin.
- Primitive plugin skips zero-sized cylinder caps.
- All Explorer Material uniform sliders have text editing mode on the right mouse click.
- Explorer Material editor supports vec2 uniforms.
- Old scenes are backed up before the Explorer scene save action.
- Explorer includes a MaterialFlow plugin for node-based material editing.
- 10_hello_image manual sample.
- 11_hello_bindless manual sample.
- platform/storage test has been added.
- materials/material_foliage.scenex animation material example.
- nodes/node_geometry.scenex geometry selection example.
- render/render_refraction.scenex render refraction example.
- material_flow_animation|anisotropy|bumpmap|cell|forward|fractal|parallax|perlin|skinned|texcoord|transparent|vertex sample scenes with MaterialFlow-based materials have been added to Engine SDK.
- Each API class has reflection methods getClassName() and getClassNamePtr().
- makeQuickCompare() function has been added to enable simple inline comparison lambdas.
- Parser::expectToken|Name() functions have been added.
- Spatial::intersection() correctly handles single Node cases.
- Control::addChild() method replaces/swaps the child control and returns the old one if it was replaced (new child) or null if it was swapped.
- ControlCombo can hide the current text with setTextEnabled() and act as simple menu.
- ControlSlider has Double and Right mouse button callback handlers.
- ControlSlider has unconstrained editing mode with the Option key pressed.
- ControlEdit can handle user keyboard events (keyboard events from other controls) with updateKeyboard() method.
- HSV color conversion has been added to the Color struct.
- An issue with a wrong number of parameters returned from String::scanf() function on empty numbers has been fixed.
- Variables with “step” or any other reserved by external function names are automatically renamed into “step_%x” by the compiler to avoid name collision on some API.
- The shader preprocessor recognizes “quiet” pragma and hides macro debug information during errors.
- The shader compiler recognizes “dynamic” pragma and avoids shader cache creation for such shaders.
- ControlRoot::setOverlayOrder() automatically fixes the issue with overlays rendering inside ControlArea.
- ControlDialog limits the number of iterations to 8 to avoid hangs.
- A vertex shader pass has been added to the MaterialShading class. This pass is performed after vertex skinning and morphing and is useful for dynamic geometry that calculates motion vectors automatically.
- All Object shaders use #assign instead of #define macro to avoid “macro has been redefined” warnings.
- attribute_position and attribute_normal shader inputs provide pre-skinned|morphed values good for procedural texture coordinate generation.
- GLTF KHR_materials_anisotropy extension support.
- HLSL access to half SSBO types.
- Global GLSL constant arrays support has been added.
- GLSL array initialization is compatible with vector types.
- MeshMaterial::Anisotropy|AnisotropyAngle parameters have been added.
- The number of SSBO buffers in Pipelines, Kernels, and Traversals has been increased to 32.
- Correct evaluation of nested shader macros without values.
- The number of barriers per barrier() call has been increased to 128.
- A random bug with incorrect parsing of some JPEG files has been fixed.
- isFinite|Normal|Inf|Nan functions are manually implemented.
- Access to SpatialTree internal buffers has been added.
- WebGPU can be initialized from a preinitialized device.
- Frame Viewport struct includes scene_time|ifps|seed|layer members.
- Correct depth bias evaluation for screen space occlusion. The banding artifact has been removed.
- HDR reflection overflow has been fixed on iOS.
- Temporal antialiasing has a better reaction to luminance changes.
- An incorrect first frame motion vector artifact has been removed.
- NodeObject has a new geometry index parameter for strict geometry selection.
- LightGlobal has a new split offset parameter for a better split distribution.
- MaterialMetallic|Specular has a PixelDepth option for random depth value for smooth depth intersection.
- MaterialMetallic|Specular has a Randomized texture coordinates option for tile-less texture sampling.
- New Apple devices have been added to system Info. A device version is also included in the system info string.
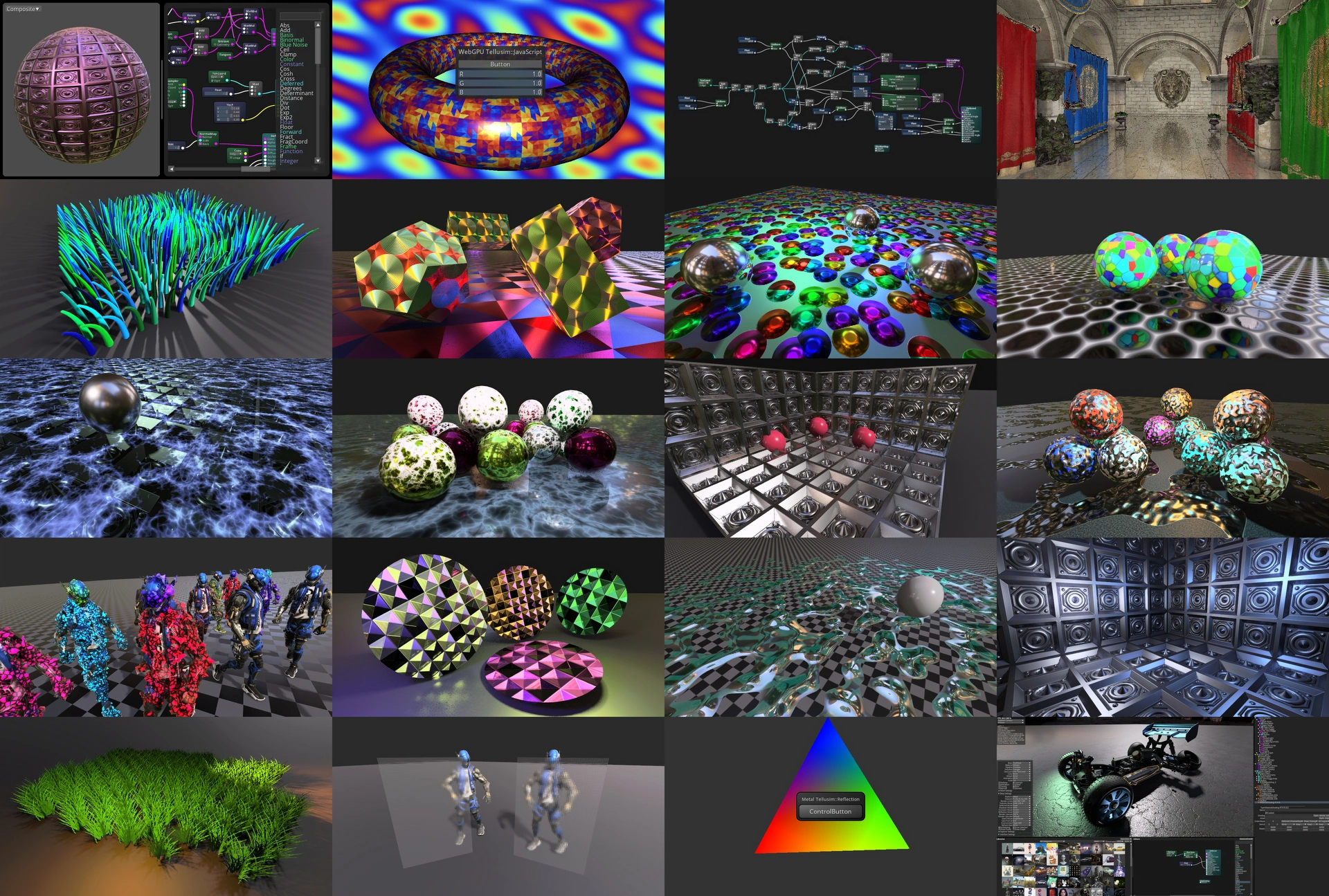
December 23, 2024
The Power of GPU-Driven Scenes
December 8, 2024
11 Hello Bindless
November 11, 2024
GPU texture encoder
November 9, 2024
10 Hello Image
November 8, 2024
SDK 40
September 25, 2024
09 Hello Controls
September 1, 2024
08 Hello Canvas
September 1, 2024
SDK 39
September 18, 2023
07 Hello Splatting
August 28, 2023
06 Hello Traversal
August 13, 2023
05 Hello Tracing
August 12, 2023
04 Hello Raster
July 31, 2023
03 Hello Mesh
July 7, 2023
Scene Import
June 25, 2023
02 Hello Compute
May 15, 2023
01 Hello USDZ
May 14, 2023
00 Hello Triangle
April 24, 2023
WebGPU Update
April 4, 2023
Tellusim Upscaler Demo
February 10, 2023
DLSS 3.1.1 vs DLSS 2.4.0
January 31, 2023
Dispatch, Dispatch, Dispatch
October 28, 2022
Tellusim upscaler
October 14, 2022
Upscale SDK comparison
September 20, 2022
Improved Blue Noise
June 19, 2022
Intel Arc 370M analysis
January 16, 2022
Mesh Shader Emulation
December 16, 2021
Mesh Shader Performance
October 10, 2021
Blue Noise Generator
October 7, 2021
Ray Tracing versus Animation
September 24, 2021
Ray Tracing Performance Comparison
September 13, 2021
Compute versus Hardware
September 9, 2021
MultiDrawIndirect and Metal
September 4, 2021
Mesh Shader versus MultiDrawIndirect
June 30, 2021
Shader Pipeline