May 31, 2025
New Tellusim Documentation
We’re happy to announce the launch of our official documentation site: docs.tellusim.com!
The new portal offers a clean, searchable hub for everything Tellusim-API references, tutorials, scripting guides, and more. Whether you’re just starting out or working on advanced features, you’ll find the resources you need to build faster and better.
Check it out now at docs.tellusim.com and let us know what you think!
April 29, 2025
SDK 44
New Engine features:
- New Engine and Core SDK release for the Apple tvOS platform.
- MuJoCo physics plugin for enhanced robotic and precision simulations.
- Introduced Inverse Kinematics plugin for Nodes and Bodies.
- GCC compiler compatibility.
- New MuJoCo Physics Plugin. Enables high-precision simulation for robotics and mechanical systems.
- New Inverse Kinematics Plugin. Jacobian-based solver supporting both positional and rotational targets. Automatically converts Slider, Hinge, and Ball joints from physics scene representations.
- Consistent Six Degrees Of Freedom joint behavior is now uniform across all physics plugins.
- Physics plugins now update joint positional parameters back into the engine for real-time state tracking.
- All motorized joints now support Servo motor mode with constant velocity across all physics plugins.
- The WebClient plugin now provides detailed information for HTTP error codes.
- Selection-by-Name Dialog has been added to the Explorer for quicker object access.
- Enhanced Joints configuration for precision and usability within the Explorer.
- Rotation and scale Explorer’s operations can now use Local, Common, or Selected centers.
- Improved JointSixDof configuration with sliders for more interactive control.
- Tellusim project file generator now supports creating Xcode projects for tvOS.
- New physics_domino, physics_ball, and physics_servo scenes have been added.
- New tests/platform/integer sample demonstrates native and emulated 64-bit integer operations.
- The tests/render/memory sample demonstrates minimal render and scene footprint.
- The tests/render/procedural demonstrates procedural object injection with full shadow support from fragment and compute shaders.
- SampleScene and SampleRender classes now maintain global self-pointers, making them always accessible during runtime.
- Device::bindBuffer() methods for sparse buffer memory allocations have been added.
- TellusimNumerical classes support variable-sized resources; storage shape is defined via templates, but computations can target smaller domains for all operations, including LU, QR, and SVD algorithms.
- TellusimSimd is now GCC-compatible. The float16_t type is encapsulated in the f16 sub-structure (replacing the unnamed struct).
- SpatialTree::dispatchIndirect() now accepts a flags argument to allow update-only BVH indirect operations.
- New RenderDraw::drawArc() method supports drawing arc shapes.
- New RenderRenderer::FlagSurfaceTextures flag applies Texture::FlagSurface to all RenderFrame textures.
- Scene rendering now allows custom shadow and reflection intersection shader functions via the SceneRenderer interface.
- Added callbacks for deferred light kernel creation and bind operations.
- Motor stiffness and damping coefficients added to all motorized joints.
- New system/TellusimtvOS header added for tvOS-specific methods.
- Cache directory name is now available through iOS and tvOS interfaces.
- New Atomici64 type added to core/TellusimAtomic.h.
- Locking behavior of SixDof joints changed; setLinear|AngularLock() now uses a mask to control axes.
- GCC compiler compatibility.
- Added support for i|umulExtended, uaddCarry, and usubBorrow GLSL functions across all platforms.
- OpenCL-based GPU core and memory queries have been added to the Info class, providing detailed GPU information on macOS.
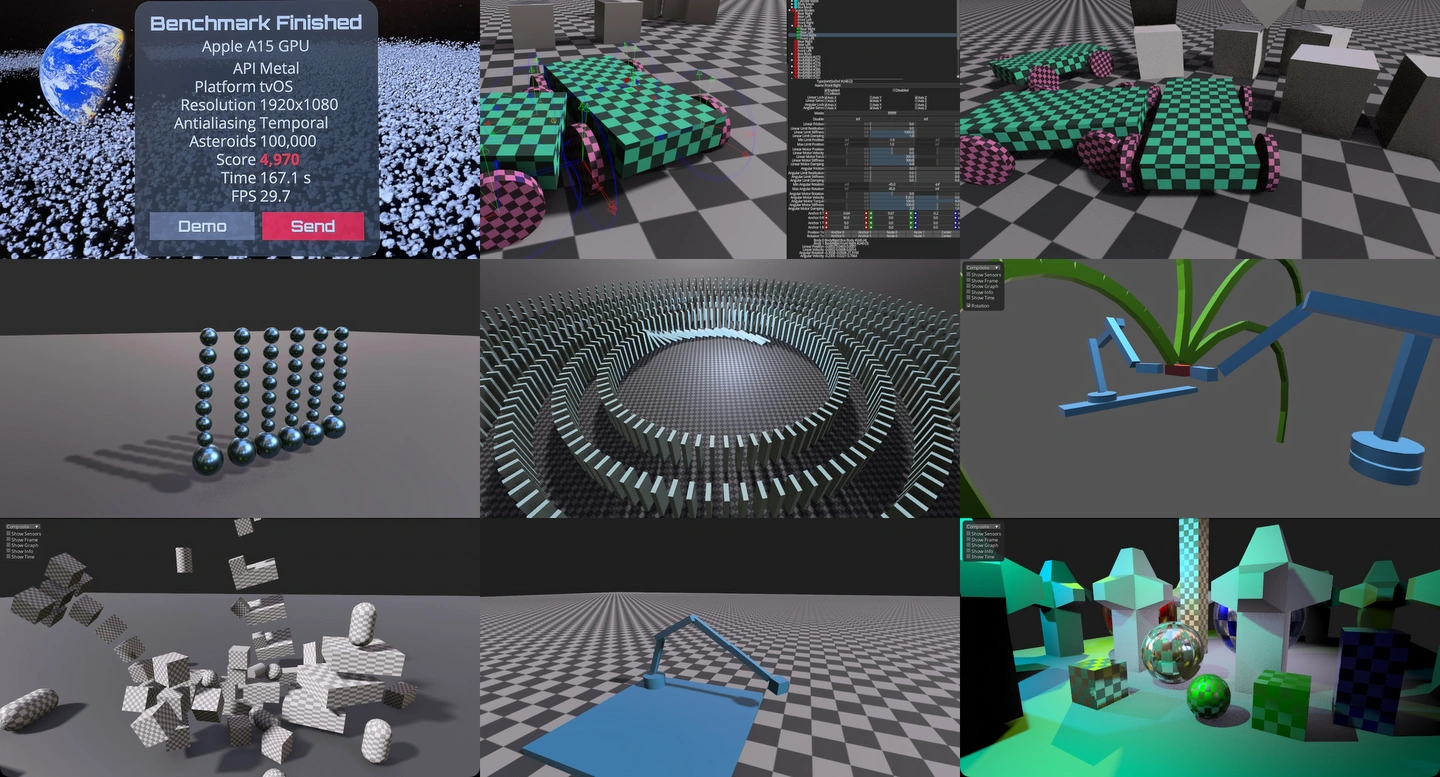
March 23, 2025
SDK 43
New Engine features:
- Added support for game controllers (Xbox, DualSense, DualShock, Nintendo Pro Controller, and generic joysticks) across all platforms.
- Improved physics engine plugin integration.
- Introduced a debug visualization API for enhanced real-time debugging.
- Implemented an Internal Color dialog for a more streamlined UI experience.
- Major improvements to the Explorer.
- Explorer has two icon sets for best icons visualization over different monitor DPIs.
- Executor and Explorer update the Controller class for automatic controller support.
- Explorer now supports multiple control info callbacks (allowing body info to be displayed within node info).
- Basic physics visualization (shapes and joints) has been added to Explorer.
- Inertia tensor information is now available in Explorer.
- All runtime parameter editors have been refactored to support right-click editing, expanding the range of editable types.
- Explorer has been migrated to the Internal Color dialog.
- Scale parameter has been added to the MeshAnimation import Explorer extension.
- The MeshPrimitive extension now correctly handles internal shapes during object editing.
- ScriptFlow and MaterialFlow extensions now use the Internal Color dialog.
- Improved geometry combination performance in the Mesh tool.
- Added 12 Hello Controller manual sample.
- Added tests/system/desktop for the Desktop class.
- Added tests/system/controller for the Controller class.
- Added new physics samples for each physics plugin (Box2D, Bullet, Jolt, PhysX), including 6dof, friction, hinge, impulse, restitution, shapes, and slider.
- Added scripts/script_node_flow_controller, a sample demonstrating flow-based virtual controller animation based on real controller events.
- Added scripts/script_node_flow_keyboard, a sample showcasing flow-based node control using window keyboard events.
- Introduced a new interface/color plugin for controls-based color dialog support.
- Box2D now supports capsule and cylinder shapes.
- The Box2D plugin has been updated to the latest Box2D library.
- The Jolt plugin has been updated to the latest Jolt library.
- All physics plugins now support engine-driven parameter updates, including friction, forces, impulses, shapes, and joints.
- Physics plugins now provide real-time velocity values to the engine.
- The default number of ControlFlow types has been increased to 512.
- ControlCallback has been added to ControlFlow to support custom color dialogs.
- PolyHaven and Sketchfab plugins now reduce preview image brightness when a modal control is present.
- ScriptFlow now includes guards to prevent assertion failures caused by invalid parameters (e.g., getting a child by index).
- The ControlFlow node dialog now displays the current node parameter value.
- The number of Tellusim::Function<> template arguments has been increased to 9.
- ControlRoot now includes a mouse offset parameter, allowing callback handlers to evaluate control offset/scale values when embedded within a ControlArea.
- Added a ‘disabled modal state’ option to ControlRoot, allowing modal focus without dimming other controls.
- Introduced new Mesh|BrepNode|Node|ObjectNode|Material::findChild|getChild() methods that accept literal name arguments.
- Introduced a movable flag for ControlDialog to restrict movement.
- Added InsideCallback to ControlRect for non-rectangular control shapes.
- Added MatrixNxM::outer() product operator.
- Implemented math operations between MatrixNxM types, including addition, subtraction, and scalar multiplication.
- RenderDraw now supports debug primitives visualization, including lines, quadratics, cubics, arrows, rectangles, quads, circles, boxes, spheres, cylinders, and capsules.
- Added a bias parameter to RenderDraw, allowing controllable depth overlap during rendering.
- BodyRigid axes locks are now controlled by BodyRigid flags instead of dedicated methods.
- Added BodyRigid::FlagKeepAwake that prevents Body from sleeping.
- Added BodyRigid::addForce(), addTorque(), addLinearImpulse(), and addAngularImpulse().
- BodyRigid::updateMass() now recalculates body mass and inertia during the next update.
- Added control over maximum linear and angular velocities for BodyRigid.
- Each SceneGraph entity now includes is*Updated() flags for better interoperations.
- Game Controller support has been added for Windows, Linux, macOS, Android, and iOS. On Windows, the system uses GameInput if available, falling back to XInput by default.
- The quat_to_mat4x3() function has been added to SceneMath.shader
- The get_body_transform() function has been added to SceneBodies.shader
- The get_num_graph_nodes() and get_graph_base_node() functions have been added to SceneGraphs.shader
- Introduced the base_scene_body member to the RenderFrame structure for accessing all body parameters.
- Tangent-space generation performance dramatically increased for meshes with same normals (giant planes).
- PrefixScan and RadixSort now correctly detect the presence of FlagIndirect for dispatchIndirect() operations.
- Corrected joint body assignment during all scene clone operations (nodes, bodies, graphs, scenes).
- Joystick events have been added to the Android activity.
- Corrected window and DialogMenu icon creation on Windows.
- Improved retina-aware DialogMenu icon rendering on macOS.
- New macOS devices have been added to Info class.
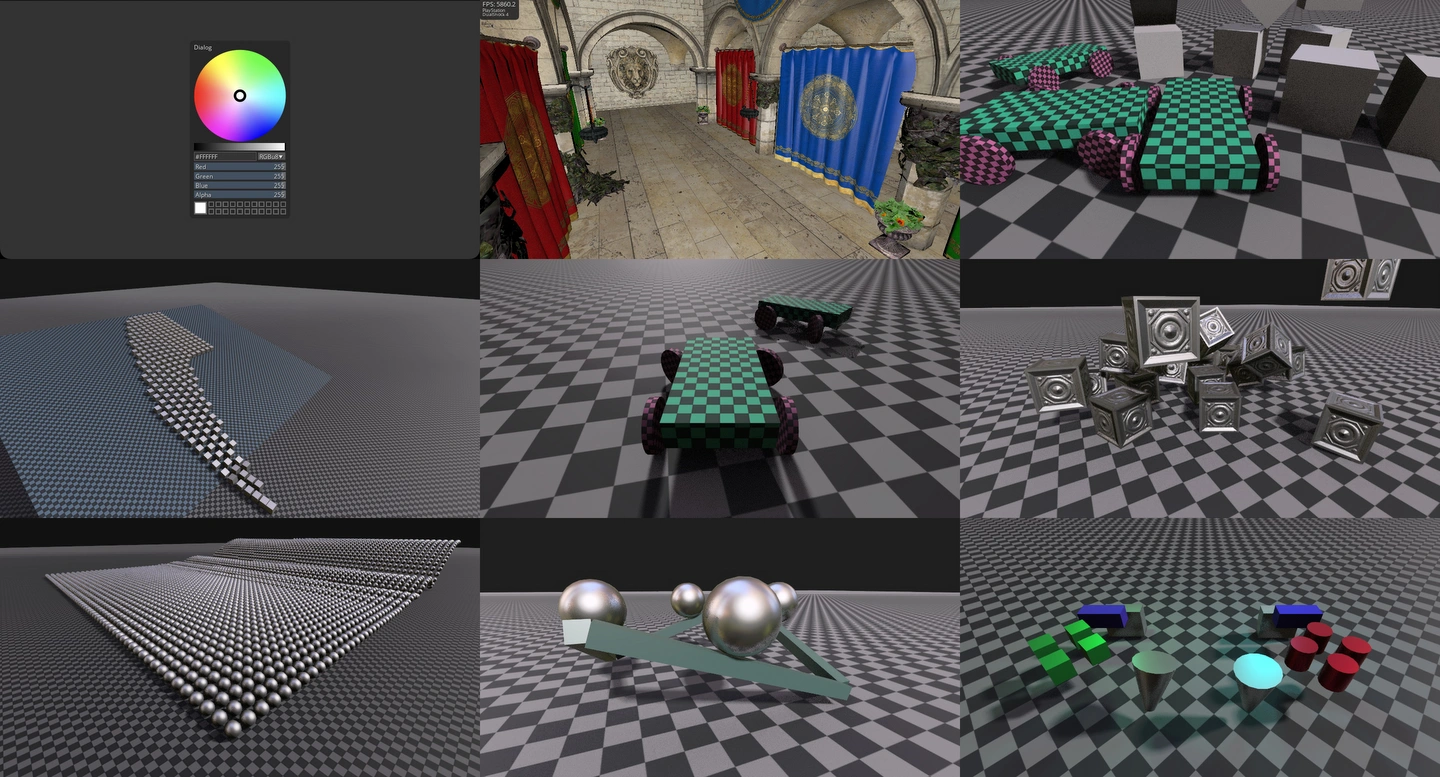
February 23, 2025
SDK 42
January 20, 2025
SDK 41
December 23, 2024
The Power of GPU-Driven Scenes
December 8, 2024
11 Hello Bindless
November 11, 2024
GPU texture encoder
November 9, 2024
10 Hello Image
November 8, 2024
SDK 40
September 25, 2024
09 Hello Controls
September 1, 2024
08 Hello Canvas
September 1, 2024
SDK 39
September 18, 2023
07 Hello Splatting
August 28, 2023
06 Hello Traversal
August 13, 2023
05 Hello Tracing
August 12, 2023
04 Hello Raster
July 31, 2023
03 Hello Mesh
July 7, 2023
Scene Import
June 25, 2023
02 Hello Compute
May 15, 2023
01 Hello USDZ
May 14, 2023
00 Hello Triangle
April 24, 2023
WebGPU Update
April 4, 2023
Tellusim Upscaler Demo
February 10, 2023
DLSS 3.1.1 vs DLSS 2.4.0
January 31, 2023
Dispatch, Dispatch, Dispatch
October 28, 2022
Tellusim upscaler
October 14, 2022
Upscale SDK comparison
September 20, 2022
Improved Blue Noise
June 19, 2022
Intel Arc 370M analysis
January 16, 2022
Mesh Shader Emulation
December 16, 2021
Mesh Shader Performance
October 10, 2021
Blue Noise Generator
October 7, 2021
Ray Tracing versus Animation
September 24, 2021
Ray Tracing Performance Comparison
September 13, 2021
Compute versus Hardware
September 9, 2021
MultiDrawIndirect and Metal
September 4, 2021
Mesh Shader versus MultiDrawIndirect
June 30, 2021
Shader Pipeline